Object Oriented Programming (OOP) is the widely accepted software development method. It’s been around for decades, and it’s used to create everything from mobile apps to complex computer systems.
This comprehensive guide covers OOP fundamentals: from classes and objects to advanced concepts like abstraction, encapsulation, and polymorphism.
It provides detailed explanations with examples and illustrations, suitable for all levels of programming experience and language proficiency.
Contents
1. Definition
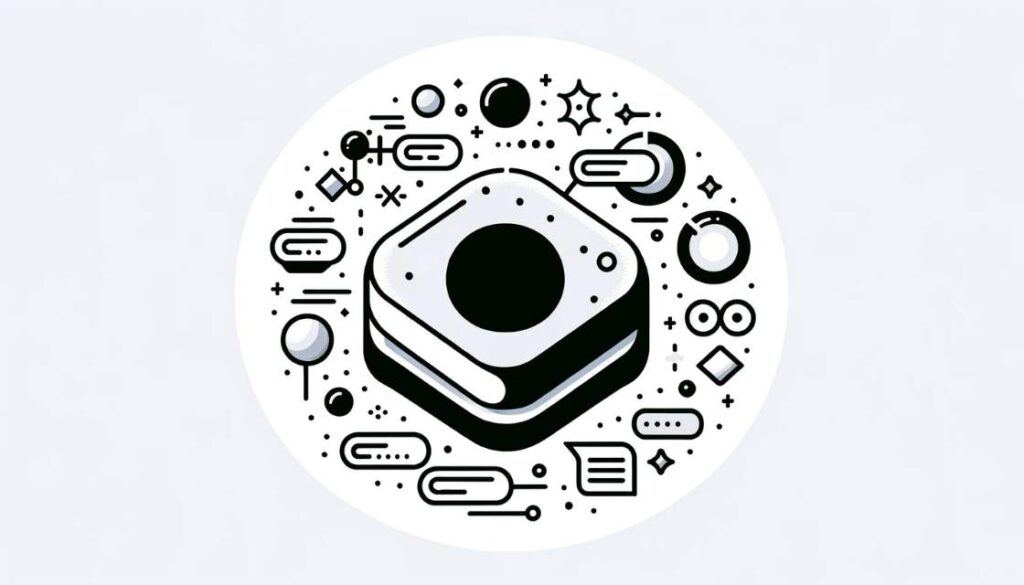
Object-oriented programming (OOP) is a powerful tool that can help solve many of today’s complex computing challenges. It’s like the wild west – there are no limits to what you can do with it.
Fundamentals of Object Oriented Programming
Regarding coding, OOP allows for greater modularity, scalability, and extensibility than traditional approaches. To gain mastery of this topic, one must first understand its fundamentals. Like the layers of an onion, each concept builds upon the previous until reaching a comprehensive understanding.
Note: At its core, object-oriented programming revolves around objects – elements containing data and logic related to their state or behavior.
Understand with an Example
Let’s compare OOP to everyday life, where an individual is an “object” with attributes and behaviors like age, height, walking, talking, and eating.
Combining multiple objects into a single program can create more complex systems capable of performing sophisticated tasks.
Using OOP effectively requires exploring basic concepts like classes, inheritance, polymorphism, and encapsulation.
2. Basic Concepts
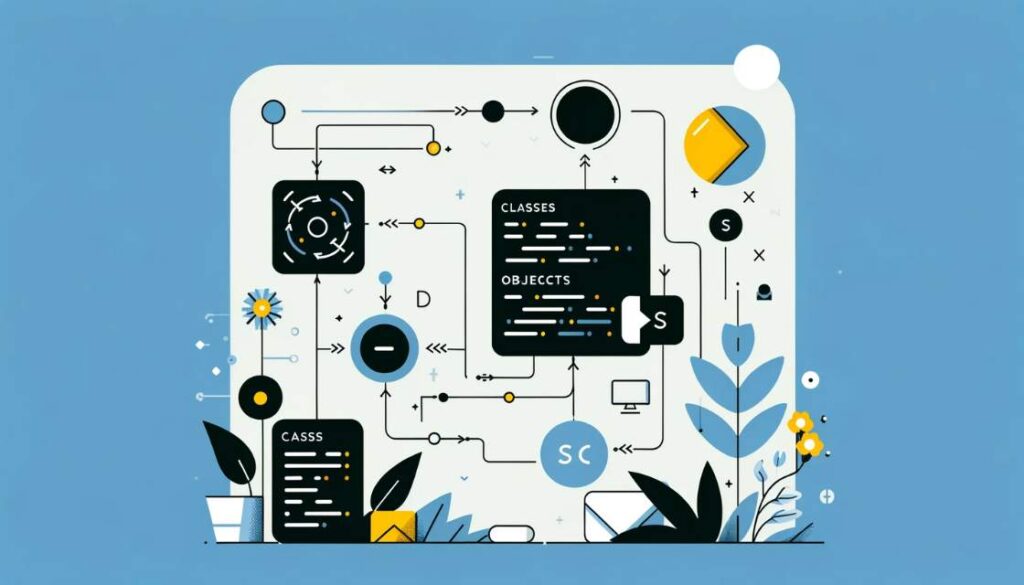
Now that we understand object-oriented programming let’s delve deeper into the basic concepts.
OOP relies heavily on the relationship between objects and classes.
Objects and Classes
An object can be considered an instance of a class; it accesses data fields and methods contained in its parent class. A class acts like a template for creating objects, providing them with structure and behavior.
Inheritance in OOP
Inheritance plays a vital role in OOP because it allows us to create new classes based on existing ones. This helps reduce code duplication by enabling developers to reuse existing code instead of writing everything from scratch.
Inheritance also allows us to easily extend our applications without having to rewrite all their core components.
Polymorphism in OOP
Polymorphism is another fundamental concept in OOP that allows developers to use different types of objects interchangeably within their programs.
Note: Objects from other classes can respond differently when given the same input or method call.
Polymorphism enables us to write more extensible programs by treating multiple objects similarly regardless of their type or origin. We gain insight into how Object-Oriented Programming works by understanding these core concepts – Classes & Objects, Inheritance, and Polymorphism.
We can utilize it effectively in designing software solutions. With this knowledge, we now look at classes and objects in greater detail.
3. Classes And Objects
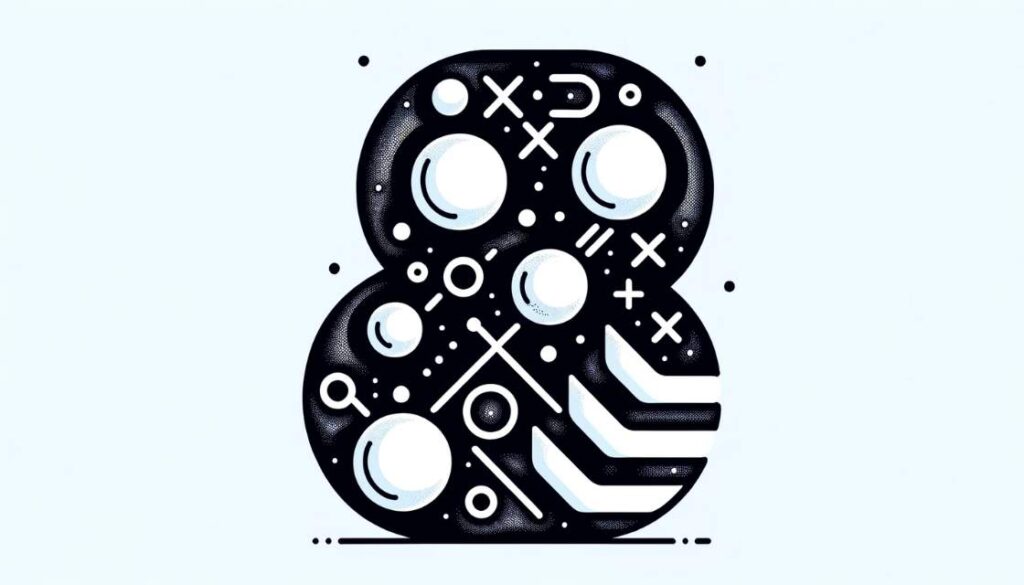
Classes and objects are the building blocks of Object-Oriented Programming. The Classes define a type of object, while an object is an instance of that class.
A class can contain methods (functions) and properties (variables). Class properties represent object attributes, while methods offer functionality to manipulate those properties.
Inheritance
Inheritance is another important concept when working with classes and objects. It allows for code reuse by allowing one class to inherit from another.
Inheritance allows children to inherit parent methods and properties, eliminating the need for duplicate code.
Subclasses also can override particular behavior inherited from their parents if they choose to do so.
Object Interaction
Encapsulation through objects enables developers to hide implementation details and only expose necessary information to users and other parts of the system.
We can focus more on how these objects will interact with each other instead of worrying about their implementations. By utilizing encapsulation, we can quickly develop robust programs as much of the underlying logic is already handled.
With that said, let’s look at encapsulation — what it is and why it matters.
4. Encapsulation
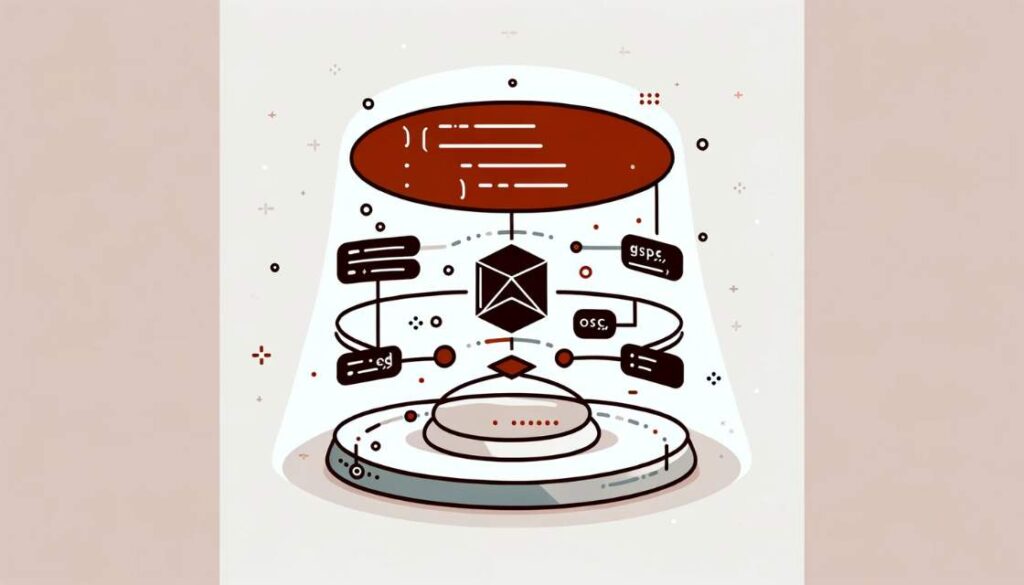
Object-oriented programming is a significant contributor to the estimated 20 million lines of code written daily.
Encapsulation is a vital concept in OOP as it enables developers to hide data and ensure effective collaboration between objects.
Wrapping the Data
Encapsulation refers to wrapping up all data related to an entity into a single unit. This protects data from unauthorized access or modification and ensures that only authorized personnel can make changes within the system.
Note: Encapsulation uses abstraction to separate the interface from implementation details, preventing users from seeing the system’s internal workings.
Understand with an Example:
To illustrate encapsulation, imagine a car where you can open the hood but not tamper with any components. It’s like having your mechanic who knows exactly how everything should look without needing to take apart each part individually.
Control Over Dependencies
Encapsulation promotes maintainable software by enhancing control over dependencies between different program components. With encapsulation, developers can easily modify or update their code without breaking other program sections.
Isolating program components such as classes, functions, and variables provides complete control over changes and simplifies maintenance in the long run.
Encapsulation adds structure and security, increases efficiency, and enhances maintainability, making it a crucial concept in OOP.
The following section will explore inheritance and polymorphism – two critical topics that further build upon this concept.
5. Inheritance And Polymorphism
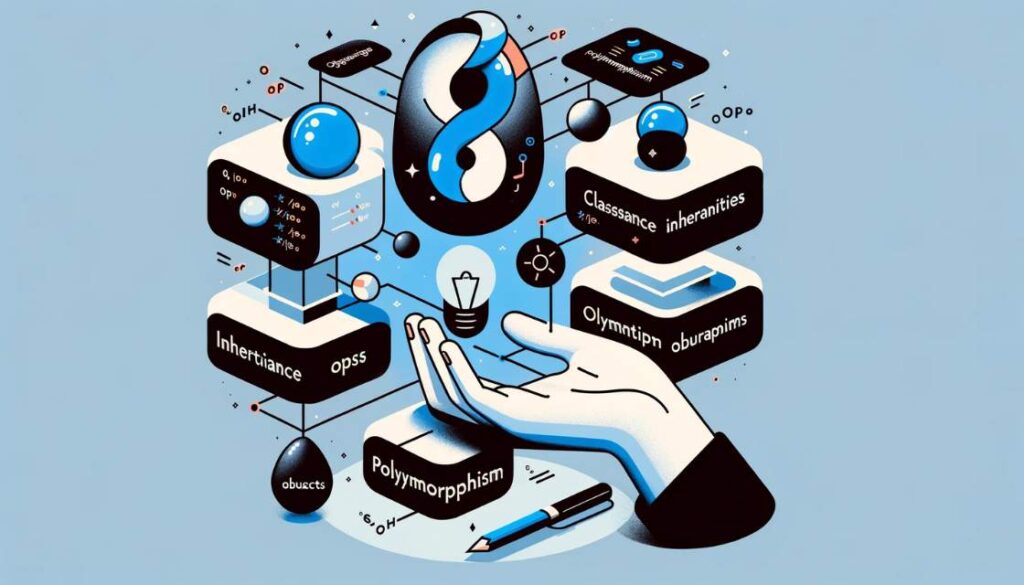
Inheritance and polymorphism are two crucial concepts in object-oriented programming.
Polymorphism and inheritance are related as it enables the use of different classes interchangeably in a program by sharing standard methods or properties.
Inheritance creates a new class that inherits all attributes and behaviors of its parent class. This allows for code reuse, which reduces development time and improves maintainability.
To demonstrate OOP principles, we can create a Vehicle subclass of a Parent class with specific characteristics like make, model, and color.
We can assign attribute values and write methods for behaviors like starting the engine. By utilizing inheritance and polymorphism, these behaviors can apply to any Vehicle type.
Abstraction helps group similar data structures under higher-level abstractions for better understanding.
6. Abstraction
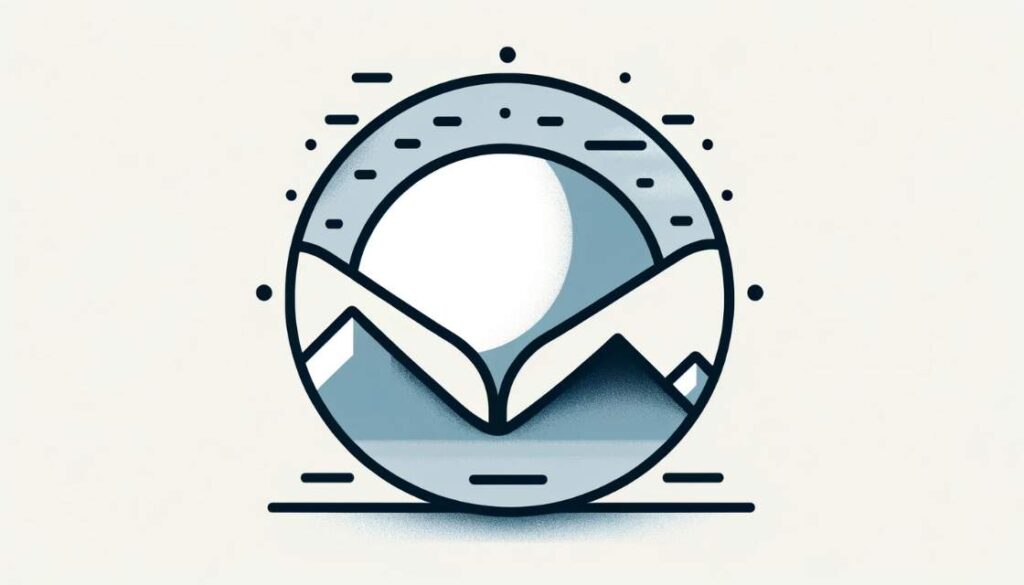
An abstraction is a powerful tool that simplifies complex problems into manageable chunks. In this way, it helps us effectively organize our code while making sure nothing superfluous gets added in.
Abstraction groups related classes with similar characteristics under one umbrella class or interface. This makes it easier to manage complexity since all methods used within a particular group can be accessed without creating individual objects for each item in the set.
It also increases reusability as different parts of the solution can be recycled across multiple projects. The use of abstractions can help us keep our code organized and make debugging much more efficient.
Abstraction encapsulates data, adding an extra layer of security by keeping sensitive information hidden within classes.
Better Flexibility and Scalability
Hiding implementations behind interfaces offers increased scalability and flexibility. It makes changes easier to apply centrally rather than throughout the program’s structure.
By creating abstractions, programmers can design secure and flexible systems to accommodate future growth.
Now let’s dive deeper into how interfaces and protocols come into play with object-oriented development.
7. Interfaces And Protocols
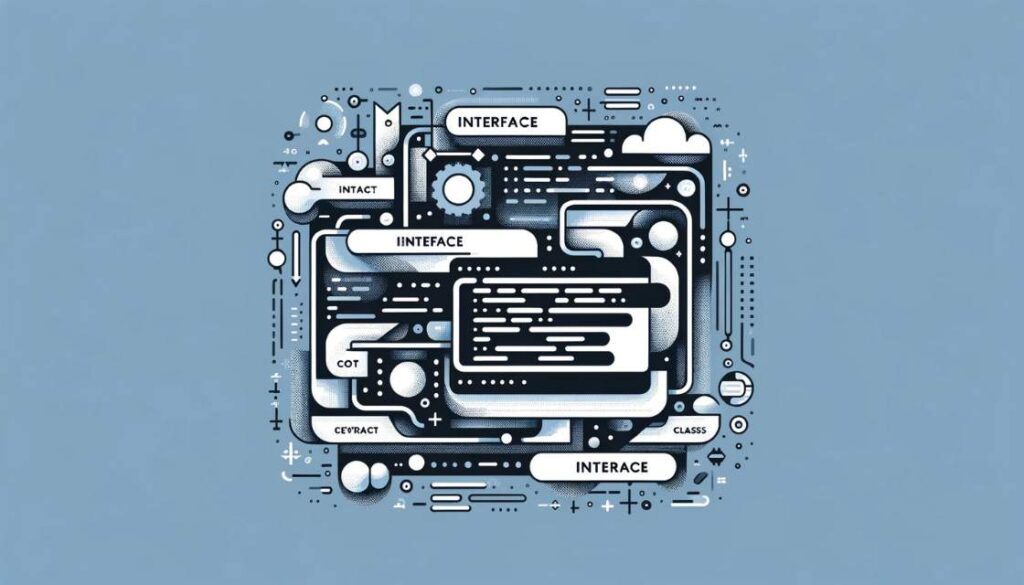
Take the example of a modern automobile. It is powered by hundreds of intricate systems and components that must seamlessly work together to keep it running smoothly.
Developers need to establish reliable interfaces and protocols to enable efficient interaction between systems, ensuring quick data exchange without errors. Interfaces and protocols are essential to this process, as they provide structure and communication between two different entities or technologies.
The use of Interfaces
Interfaces bridge users and technology, allowing them to transfer information without understanding how the system works behind the scenes. Protocols define rules on how data should be transmitted securely and reliably over networks while ensuring compatibility among different devices.
Interfaces and protocols enable seamless communication between software applications, regardless of their design or purpose.
Secure, User-Friendly System Development
Ensuring your interface is user-friendly yet secure enough to transfer sensitive data is essential when developing any system.
Clear boundaries and standard industry practices make complex tasks simpler over time.
And when creating protocols, make sure that you adhere strictly to established standards such as HTTP or HTTPS. Otherwise, your application might not be able to connect with others in its environment correctly.
Effective interfaces and protocols lead to successful error-handling techniques, ensuring maximum uptime across interconnected systems.
8. Error Handling
Error handling is an integral part of object-oriented programming. It allows developers to detect and respond to errors that occur during the execution of their code.
Errors can range from simple typos in a program’s source code to more complex issues like memory allocation failures or unhandled exceptions. Error handling helps ensure that programs run as expected, even when unexpected errors occur.
Identify the Error:
The first step towards effective error handling is understanding what errors might arise within your application.
Common sources of errors include invalid user input, network connection issues, and resource contention between different parts of the system.
Handling the Error:
Once you have identified potential sources of errors, you need to decide how best to handle them.
Error-handling techniques can involve displaying informative messages, logging detailed information, or ignoring non-critical issues.
Creating Error-Handling Logic
Consider the impact on maintainability and scalability when creating error-handling logic for your applications.
Error messages should be meaningful enough for other developers who may work with your code later down the line. While also being concise enough so that they don’t become overwhelming in large systems. With this in mind, choosing an appropriate strategy becomes much easier.
Established good error-handling practices make debugging strategies far easier and faster. It allows developers to quickly identify where problems lie and take corrective action before they affect end users.
9. Debugging Strategies
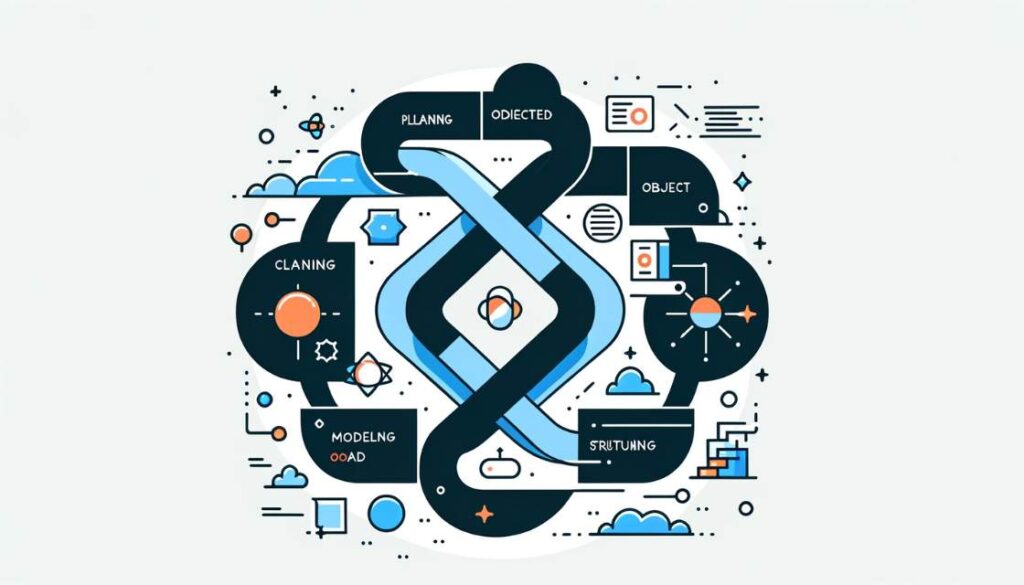
Debugging strategies are like a detective’s toolkit. With the right tools, you can solve any issue and be one step closer to object-oriented programming success.
Here is an essential list of debugging techniques that every programmer should know:
- Tracing – tracing allows you to understand where your code has gone wrong by following its trail of execution;
- Logging – logging records events during program execution so that they can be examined later;
- Breakpoints – setting breakpoints pauses the program at specific points, allowing for a more precise examination of what is happening; and
- Testing involves running test cases against the code to ensure it works properly.
When using these techniques, always remember to stay organized! Tracking errors with notes or comments will save time when identifying problems.
When done correctly, debugging strategies can make all the difference between writing effective and efficient code. Knowing how each method applies in different situations can help speed up troubleshooting and provide experience when tackling complex issues.
Now let’s explore some application examples of object-oriented programming principles!
10. Application Examples
Object-oriented programming has been used to create a wide range of applications. From video games, navigation systems to financial software, it provides developers the flexibility for dynamic and robust programs.
Airline Reservation System
One example is an airline reservation system. This program relies heavily on objects like customers, flights, airports, tickets, etc.
Developers can easily create complex features such as seat selection or flight routing by writing code that interacts with these objects. The same basic principles apply to other types of applications, too; if you can identify the parts of your application and how they interact, you can use object-oriented programming to make them work together efficiently.
Extending Existing Application
In addition to creating new applications from scratch, OOP allows developers to extend existing ones by adding custom components without reworking the entire codebase.
By encapsulating related pieces of code into objects, developers can easily add new functionality while keeping their changes isolated from the rest of the system’s operations. Such an approach is beneficial when dealing with legacy systems with hundreds or even thousands of lines of code already written.
11. Conclusion
This ultimate guide has demonstrated what makes Object Oriented Programming so powerful: its ability to bring order out of chaos by breaking down complex tasks into smaller chunks that are easier to manage.
With this knowledge, anyone who wants to build robust digital products should understand why OOP is one of today’s most popular coding techniques.
As a developer, you must stay up-to-date on the latest techniques and trends to provide your clients with optimal solutions. Just like a master artisan craftsman who perfects their craft over time.