Variables and constants are like the core building blocks of any programming language. With this comprehensive guide, you will master these concepts of programming.
So let’s dive into the guide!
Contents
1. Difference Between Variable and Constant
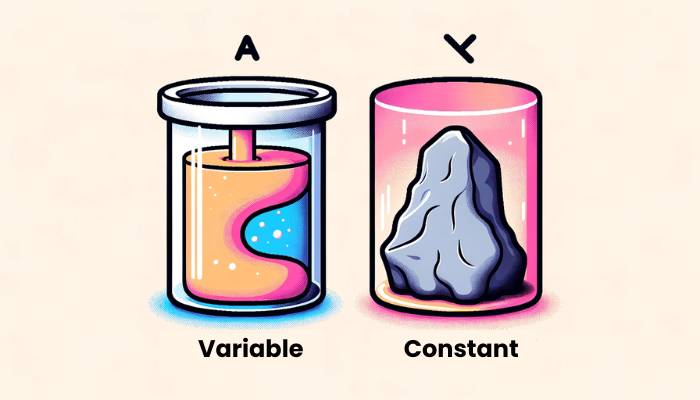
Variables and constants are integral parts of programming. Understanding how these concepts work is essential for progressing in your coding journey.
I. Variables
A variable is an object whose value can change over time or between different contexts. Consider variables as containers that store information like numbers, words, images, etc.
Naming Convention:
Variables can be named anything you want them to be – it all depends on what type of data they contain and how you plan to use them.
II. Constants
On the other hand, constants are values that remain unchanged throughout a program’s execution process. These objects stay constant no matter what happens in the code elsewhere.
Constants provide stability by anchoring programs down so they don’t become too chaotic and unpredictable; after all, having some stable elements makes debugging much more effortless!
With this knowledge in mind, let’s explore the different types of variables and constants available in programming languages today.
2. Types Of Variables And Constants
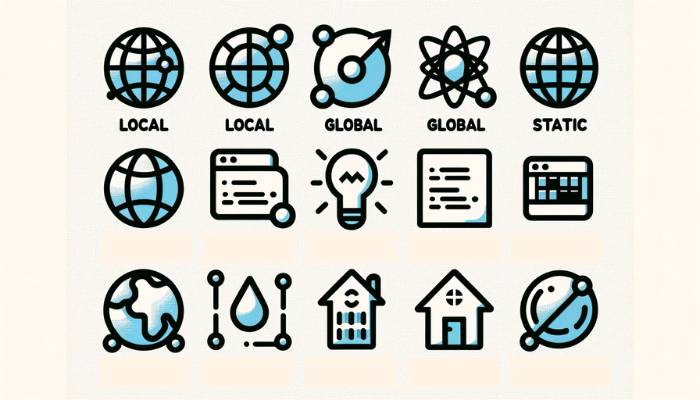
Variables and constants are the fundamental building blocks of programming. They come in many sizes, each serving a different purpose.
I. Primitive Data Type
The first variable is a primitive data type, like numbers or strings. Primitive data types can only store one value at a time, such as an integer or text string.
These values cannot be changed once assigned and are often used to represent simple information.
II. Reference Data Type
The second variable type is reference data types, which include objects and arrays. Reference data types can contain multiple related items, such as a list of names or things with various properties.
Unlike primitive data types, reference data types are mutable, meaning their content may be modified after being declared initially.
This makes them powerful tools for managing more extensive amounts of complex data within programs.
III. Constant Values
Finally, constants are unique that have fixed values throughout the lifespan of a program.
Constants allow for code readability by clarifying what specific values should not change during execution – which would otherwise require extra logic checks when dealing with regular variables.
IV. Uses of Constants
From setting error codes to defining physical constants like pi (π) or epsilon (ε), there are countless uses for constants in programming languages today.
With these core concepts in mind, we can look further into naming conventions for variables and constants.
3. Naming Conventions For Variables And Constants
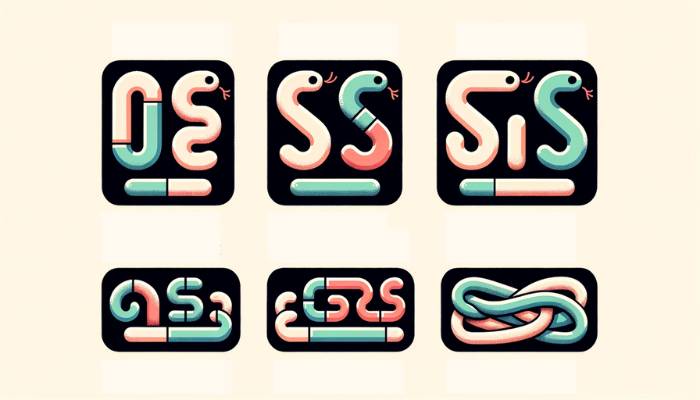
Naming conventions are essential when it comes to programming with variables and constants. They help us make sense of the code, allowing us to identify values quickly and accurately.
Variables and constants should be descriptive yet concise and follow a consistent format throughout the program.
Note: When naming variables or constants, you’ll want to avoid using confusing terms such as ‘int’ or ‘str.’ Instead, choose words that clearly describe what the variable is used for.
For example:
If you’re writing code for a calculator application, your variable names could include ‘input1’, ‘input2’, and ‘result.’ These explicit identifiers will help other developers read and understand your code more efficiently.
I. Lowercase Letters
Using lowercase letters in your variable names is also a good idea. This makes distinguishing between different variables at a glance easier because capitalized words typically have special meanings in programming languages (like Python).
Following this simple guideline can save you time when debugging later on down the road.
By taking some extra time upfront to correctly name our variables, we create an organized structure within our programs and an easy-to-understand set of rules from which anyone looking at our code can learn.
With that in mind, let’s examine how scope affects variables and constants next.
4. Scope Of Variables And Constants
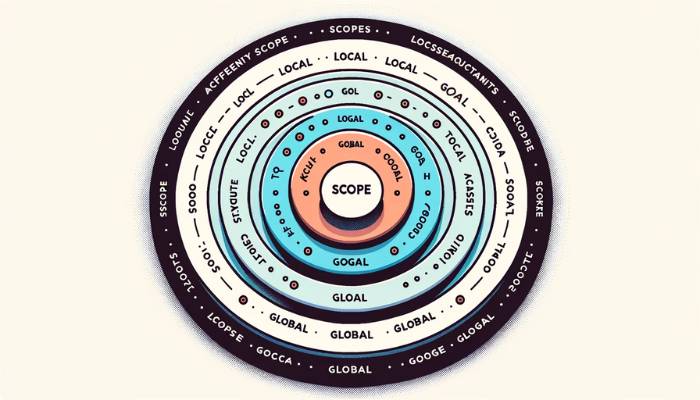
Variables and constants are essential components of programming. They allow us to store, organize, and manipulate data according to our needs.
But what exactly is the scope of variables and constants?
A variable’s scope determines where its value can be accessed or modified in a program.
I. Local Scopes
The variable can only be used within that instance in local scopes.
For example: If a variable is declared inside a loop, it cannot be used outside it.
II. Global Variables
Global variables have broader access; they can be accessed anywhere in the program and remain unchanged throughout execution.
Constants also have limited scope – their values do not change once defined, though, unlike global variables, these values may differ depending on where they are located.
III. Understanding the Scope
As we explore different types of variables and constants available to us as programmers, understanding their scope plays a vital role in ensuring correct program behavior. Awareness of when certain pieces of code can be reused or where specific information resides can save time while providing greater control over our programs‘ output.
By becoming familiar with the differences between local and global scopes and those associated with constants, we gain valuable insights into how best to use them to create powerful applications efficiently.
Let’s move on to declaring variables!
5. Declaring Variables
Declaring variables is integral to programming, allowing us to store data and information for later use. Variables can be declared in any language, but the syntax may differ depending on your language.
Declaring a variable means creating it to be used within your program. It’s important to remember that declaring a variable does not assign a value; instead, it simply creates space in memory where values assigned later will be stored.
Here are three critical points about declaring variables:
- A type is needed when declaring the variable, which tells the computer what data the variable holds. Common types include integers, strings, floats, and booleans.
- Each variable must have a unique name that cannot conflict with other names or keywords in your chosen programming language.
- The variable’s scope should also be considered when creating them – this determines how long they exist before being destroyed by the system or removed from memory after its use.
Now we will move on to assigning values to those variables already declared!
6. Assigning Values To Variables
After declaring a variable, the next step is to assign values to it. This process is like packing for a trip: you need to decide what items are necessary and where they should be stored.
Similarly, when assigning values to variables, we must first determine which information needs to be saved.
I. Understand with an Example
To illustrate this concept further, let’s look at an example of how someone might pack their suitcase for a weekend getaway. They may bring clothes, toiletries, electronics, books, or other items to make their stay more enjoyable.
Each item has its place in the suitcase; the clothing goes into one compartment while the charger is placed in another pocket. In programming terms, these pockets represent different locations within the computer’s memory where data can be stored safely until retrieved later.
Item | Memory Location (Pocket) | Description |
---|---|---|
Clothes | Main Compartment | Clothing items such as shirts and trousers needed during the trip |
Toiletries | Top Compartment | Soaps and shampoos required by travelers |
Electronics | Side Pocket | Chargers and mobile phones used on vacation |
Books | Bottom Compartment | Novels or magazines read throughout journey |
Associating certain pieces of information with specific memory locations allows us to access them quickly and easily whenever needed.
As demonstrated through our analogy of packing a suitcase for a weekend getaway, we can use similar principles when assigning values to variables in programming languages. Determining which data goes into which “pocket” to be re-accessed later if necessary.
Understanding how these concepts work together helps programmers create efficient applications that run smoothly without hiccups. With this knowledge, you’re now ready to explore accessing stored values from variables.
7. Accessing Stored Values In Variables
Once we have stored values in variables, we must access them. This is usually done through operators and symbols that can manipulate and compare values within a variable.
Note: Operators are used to making decisions and executing operations within programs. They can also help us extract data from variables or combine multiple pieces of data into one value.
The most common operator types include arithmetic, comparison, logical, assignment, bitwise, and string operators.
Operators | Description |
---|---|
Arithmetic Operators | Arithmetic operators perform basic mathematical calculations such as addition (+), subtraction (-), multiplication (*), division (/), modulus (%), power (**), and root (#). |
Comparison Operators | Comparison operators determine if two expressions hold true or false by evaluating whether they’re equal (==), less than (<=), or greater than (>=). |
Logical Operators | Logical operators allow more complex decision-making with their ‘and’ (&&) and ‘or’ (||) functions. |
Assignment Operators | Assignment operators assign a new value to an existing variable (=). |
Bitwise Operators | Bitwise operators work on binary numbers; finally. |
String Operators | String operators combine strings using the plus symbol (+). |
These different types of operators make accessing the stored information inside our variables easy. With careful thought into setting up our code structure, accessing this data quickly becomes easy.
Here’s a guide I found that will help you understand these concepts with solved examples.
8. Initializing Constant Values
‘The Only Thing That is Constant is Change’ – Heraclitus, circa 500 B.C.E. This rings true in programming languages.
Constant values are immutable and unchanging, but they must still be initialized before they can be used effectively within code. To help you get started with initializing constants, here’s a list of the 3 main things to consider:
1. Naming – Constants should have descriptive names that make them easily identifiable when reading your code.
2. Assigning values – Assign the correct data type for each constant to work correctly with the rest of your code.
3. Scope – Define where each constant can be accessed within your program, as variables or constants declared outside functions may not always be accessible inside them.
Initializing constants requires some forethought to ensure their stability throughout your program’s life cycle, which makes it an essential part of software engineering best practices.
Let’s look at how we can use operators with variables and constants.
9. Using Operators With Variables And Constants
Writing code that uses variables and constants can be tricky. To do it correctly, you’ll need to understand the various operators available in programming languages.
Each operator type has a unique set of symbols to be used when writing code.
Becoming familiar with these symbols is essential so your code will work properly when executed.
For example:
- Arithmetic operators use plus signs (+), minus signs (-), asterisks (*), etc.
- Comparison operators use greater than (>) and less than (<) symbols
- Logical operators use AND (&&) and OR (||) symbols, etc.
The next step is learning debugging tips for troubleshooting errors related to working with variables and constants – an essential skill every programmer needs to know!
10. Debugging Tips For Troubleshooting Variable Errors
Troubleshooting variable errors can be a daunting task, especially for beginners. Fortunately, several debugging tips and tricks can help simplify the process.
From checking syntax to understanding the scope, these steps will provide a practical roadmap for eliminating mistakes.
I. Double Check your Code
Firstly, it is essential to double-check your code’s syntax or grammar before running it; this means making sure all of the brackets, parentheses, semicolons, commas, etc., are in their proper places.
Syntax errors may appear nonsensical when you run your program, so reviewing the code beforehand will save time.
Additionally, if applicable, use comments within the code to describe what each section does – this makes future edits easier and allows others who view your work to understand its intentions more quickly.
II. Interdependent Variables
The next step involves examining any interdependent variables that could interfere with one another’s values during execution.
This includes reviewing whether two separate variables have been assigned identical names, which would cause confusion (and often result in unintended consequences).
It also implies assessing how changes made elsewhere might affect any given variable’s value – it is essential to fully comprehend how different pieces of data interact with each other.
To ensure accuracy, identify where a particular variable has been declared within your program and note if/where its value has changed throughout its lifespan.
III. General Principles
Finally, familiarize yourself with general principles like lifecycle rules concerning local versus global scope. And initialization techniques such as immediate assignment statements and declarations are done through function calls or method invocations.
Knowing these concepts helps to prevent a range of potential pitfalls while coding: from uninitialized variables being used without warning to unexpected results due to improper reference assignments.
By closely following these strategies, you should eventually gain greater control over debugging complex programs involving variables and constants!
IV. Testing Small Code Portions
Try to test small portions of your code before running them all together.
This way, bugs can be easily isolated by pinpointing exactly where things go wrong rather than having inconsistent outcomes across multiple instruction lines.
11. Conclusion
In conclusion, mastering using variables and constants in programming is an essential step for any beginner programmer.
You can add complexity and flexibility to your programs with a basic understanding of declaring, accessing, and manipulating these two data types. As with all aspects of coding, practice makes perfect.
I. Personal Experience
To illustrate the point above, I recently had trouble debugging some code that involved constants; it took me several hours to track down the issue and correct my mistake.
If I had taken more time to name my constants correctly and double-check their values before including them in the program, I could have saved myself a lot of frustration.
By taking the time to understand variables and constants now, you’ll be able to avoid similar issues in the future – making programming much smoother and more accessible overall.